How Do You Make A Youtube Bot?
How To Build a Youtube Comment Bot with Python
Posted past Marta on April 20, 2021 Viewed 15285 times
This tutorial will show you how to build a youtube comment bot step by step using Python and the Selenium library. Building your youtube comment bot can aid you abound your youtube audience as interacting with other channels will bulldoze traffic to your own channel.
Besides, building a youtube bot is an enjoyable thing to program. You will larn how to plan the bot to navigate the youtube site: open up videos, click buttons and enter comments. Furthermore, you could apply all selenium tricks in this tutorial to build bots for other websites.
Permit's dive in!
Overview
Our Youtube bot will simulate existence a regular user. It volition open up the browser, log in on youtube and enter any search term yous like on the search expanse. So it will start opening each of the resulting videos, get out a message and get back and click on another video, merely like a user would do.
When running a selenium bot confronting a site like Youtube, it'due south important to simulate man behavior to avoid being detected and banned. Therefore, calculation pauses when typing or clicking is crucial to replicating what a user would do.
Withal, different an actual person, the bot doesn't go tired and will keep writing messages for as long as you wish. And for as long as It goes undetected. Permit'southward see step by step how to program the bot to carry on this task.
Stride #1: Install Selenium
The first step to build a Python Youtube comment bot is installing the selenium library. You caninstall the library using thepip
tool, which manages all python installed libraries.
Y'all can install selenium executing the following control from your last:
To use selenium yous also need a driver, which is basically a browser. You could simply use your already installed browser or download a driver. I would recommend downloading a driver to avert messing up your browser settings. You lot can download a driver following the link below:
- Chrome: https://sites.google.com/a/chromium.org/chromedriver/downloads
- Edge: https://developer.microsoft.com/en-united states/microsoft-edge/tools/webdriver/
- Firefox: https://github.com/mozilla/geckodriver/releases
- Safari: https://webkit.org/blog/6900/webdriver-support-in-safari-x/
Brand a notation where you save this file, you volition demand it in the following steps.
Step #ii: Open Youtube site
By at present, you should have selenium installed and the driver file. Fourth dimension to start adding some code to our bot. The first step is opening the youtube site: www.youtube.com. To open youtube with your bot, yous will need to create a new python script and add the following code:
from selenium import webdriver browser = webdriver.Chrome('./chromedriver') browser.go("https://www.youtube.com")
Delight annotation that your python script and the chrome driver file should be in the same folder.
Step #3: Login with a username and password
The next stride is signing in to Youtube. Signing in consists of five simple tasks:
- Typing your email
- Clicking adjacent
- Typing the password
- Click next
- Sometimes clicking on confirm, although this footstep is not ever needed.

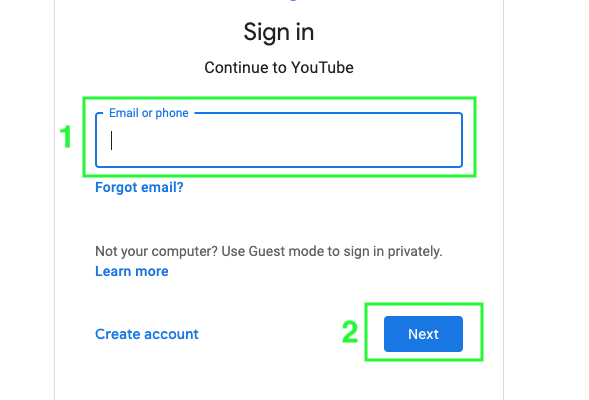
In the lawmaking snippet beneath, we define a function that volition behave out all actions to a higher place in our bot browser. We volition paste this code in our bot script between the imports line, the kickoff line, and line 3.
.login_with_username_and_password()
function
def login_with_username_and_password(browser, username, password): # Type email email_input = browser.find_element_by_css_selector('input[type=email]') email = username for letter of the alphabet in email: email_input.send_keys(letter) wait_time = random.randint(0,1000)/yard time.sleep(wait_time) # Click next next_button = browser.find_elements_by_css_selector("button") fourth dimension.sleep(2) next_button[2].click() time.sleep(2) # Type countersign password_input = browser.find_elements_by_css_selector('input[type=password]') password = password for letter of the alphabet in password: password_input.send_keys(alphabetic character) wait_time = random.randint(0,1000)/thou time.sleep(wait_time) # Click adjacent next_button = browser.find_elements_by_css_selector("push") fourth dimension.sleep(2) next_button[1].click() # Click Confirm confirm_button = browser.find_elements_by_css_selector("div[role=button]") time.sleep(2) if(len(confirm_button)>0): confirm_button[1].click()
The code above will first classify the target component using a CSS selector. In Selenium y'all tin can exercise then using two functions:
-
browser.find_elements_by_css_selector('')
-
browser.find_element_by_css_selector('')
The critical deviation between these two methods is that the start method returns a listing containing all elements in the DOM document matching the CSS Selector. However, the 2d method only returns ane element, the first matching element.
Once we accept located our target component, we tin perform an action like clicking, sliding, typing, etc. In this tutorial, we will mostly click and type.
To click nosotros volition use the .click()
method:
next_button = browser.find_elements_by_css_selector("button") next_button[two].click()
And to type we volition use the .send_keys()
method:
password_input = browser.find_elements_by_css_selector('input[type=password]') password_input.send_keys("any string can become here")
Step #iv: Enter a search term
Next, we define how to enter a search term into the search box. To practice and so, nosotros volition create a function passing in the browser and the search term. You tin paste this function in your python script under the previous function.
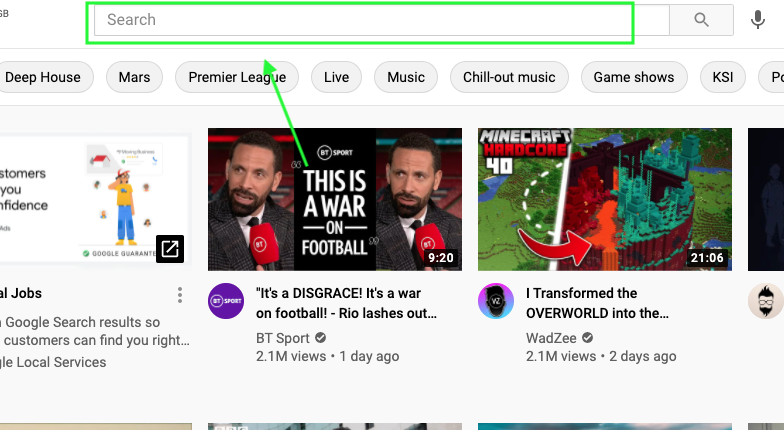
.enter_search_term()
function
def enter_search_term(browser,search_term): # Enter text on the search term search_input = browser.find_element_by_id("search") for letter in search_term: search_input.send_keys(letter) wait_time = random.randint(0,thou)/1000 time.sleep(wait_time) search_input.send_keys(Keys.ENTER)
As mentioned in the previous footstep, nosotros will first locate the search input component in the DOM document. In this instance, we will utilize the method .find_element_by_id("search")
passing every bit statement the id of the target component:
search_input = browser.find_element_by_id("search")
And so enter the search term, letter by letter using the method .send_keys()
:
for alphabetic character in search_term: search_input.send_keys(letter) # Quick pause between letter to replicate human behavior wait_time = random.randint(0,1000)/1000 time.sleep(wait_time)
Footstep #five: Click on a video
Later on entering our search term, youtube will display a listing of video suggestions based on our search. Therefore all we need to do at this point is selecting i of the videos and click. We could select one random video, or alternately select the first ane, and follow the list club.
thumbnails = browser.find_elements_by_css_selector("ytd-video-renderer") for alphabetize in range(one,6): thumbnails[alphabetize].click()
In the code snippet above, we utilise the .find_elements_by_css_selector()
method to select all videos listed on the page. And then, starting on the showtime one, click sequentially on each video.
Step #6: Enter a comment
Finally, the crucial step, entering a comment. Once our browser opens the video folio, we can insert our comment. To enter a comment, we demand to do the following:
- Motion to the comment input field
- Click on the component, so it gets the focus.
- Type our comment
- Press the "Comment" push button

In the lawmaking snippet, nosotros ascertain how to perform all the deportment in a higher place:
- Starting time, finding the target chemical element, in this instance the comment input field.
- Adjacent we volition grouping the adjacent actions: move, click and type in an action chain, using the
ActionChains
form. - And lastly our bot will click confirm.
.enter_comment()
role
from selenium.webdriver.mutual.action_chains import ActionChains def enter_comment(browser, annotate): comment_input = browser.find_element_by_css_selector("ytd-annotate-simplebox-renderer") entering_comment_actions = ActionChains(browser) entering_comment_actions.move_to_element(comment_input) entering_comment_actions.click() for letter of the alphabet in comment: entering_comment_actions.send_keys(letter) wait_time = random.randint(0,grand)/1000 entering_comment_actions.suspension(wait_time) entering_comment_actions.perform() time.sleep(1) send_comment_button = browser.find_element_by_id("submit-button") send_comment_button.click()
As before, in order to closely mimic homo behaviour, we will insert a milliseconds intermission later on typing each letter of the alphabet( line 13 and 14)
Step #seven: Get back
Then far our bot has opened a video and enter a comment. Nosotros volition need to go dorsum to the video list and click on the next video. Nosotros can achieve that easily press the back push button in the browser. In Selenium, we can get back to the previous page using the following code:
browser.execute_script("window.history.go(-1)")
The code in a higher place volition open the previous url in the browser history.
Step #8: Put it all together
At this indicate, we have some code in our bot script containing functions that volition log in to youtube, enter a search term in the search box and enter a annotate. The terminal pace is connecting all the pieces.
The code below utilizes all functions created in a higher place and volition open youtube and log in. Then nosotros will employ a set of search terms, and for each search term our bot will: enter the search term, and get out a comment on the first v video listed. And lastly, it volition close the browser. Please note you tin find the .click_on_agree_and_signin()
function defined below.
browser = webdriver.Chrome('./chromedriver') browser.get("https://www.youtube.com") # Click Agree and Sing In click_on_agree_and_signin(browser) # Sign In login_with_username_and_password(browser, "your_username_here", "your_password_here") all_search_terms =['make money online','online marketing'] for search_term in all_search_terms: enter_search_term(browser, search_term) time.sleep(2) thumbnails = browser.find_elements_by_css_selector("ytd-video-renderer") for index in range(i,vi): thumbnails[index].click() fourth dimension.sleep(6) enter_comment(browser,"your comment here") browser.execute_script("window.history.go(-1)") thumbnails = browser.find_elements_by_css_selector("ytd-video-renderer") fourth dimension.sleep(1) browser.close()
.click_on_agree_and_signin()
function
def click_on_agree_and_signin(browser): agree_button= browser.find_element_by_css_selector('push') fourth dimension.slumber(two) agree_button.click() signin_buttons= browser.find_elements_by_css_selector('yt-push button-renderer') time.sleep(six) # Wait longer so the message pops up while(len(signin_buttons)== 0): signin_buttons= browser.find_elements_by_css_selector('yt-button-renderer') time.sleep(1) signin_buttons[1].click()
Source Code
Source code available hither.
Decision
In decision, you learn how to build a youtube comment box using python and selenium in this tutorial. We accept covered all steps the bot should perform from login, and then searching video, and so entering a comment using selenium. Additionally, this tutorial provides excellent examples of using selenium to automatically navigate a website, which you could apply to automate whatsoever other dull browser task.
I promise you savor this commodity, and thank you so much for reading and supporting this blog!
More Interesting Articles



Source: https://www.hellocodeclub.com/youtube-comment-bot/
Posted by: husebycoust1990.blogspot.com
0 Response to "How Do You Make A Youtube Bot?"
Post a Comment